Contents
- 1. Using the CSS animation shorthand
- 2. Naming the animation
- 3. Specifying the duration
- 4. Manipulating the animation pace
- 5. Defining the CSS animation delay
- 6. Specifying the number of animation cycles
- 7. Modifying animation direction
- 8. Styling an inactive animation
- 9. Is the animation running?
- 10. Browser support
Using the CSS animation shorthand
By using the CSS animation
property, you can animate other properties:
.animated {
-webkit-animation: go 2s infinite; /* Chrome, Safari, Opera */
animation: go 2s infinite;
}
It is actually a shorthand for eight subproperties:
animation-name
animation-duration
animation-timing-function
animation-delay
animation-iteration-count
animation-direction
animation-fill-mode
animation-play-state
We will be explaining each animation subproperty separately in the following sections.
The syntax rules for using the CSS animation
property are simple:
animation: name duration timing delay iteration direction fill play;
As you're using the shorthand, there's no need to list the subproperties – you just need to define the values you need. You don't need to use all of the subproperties, but animation-name
and animation-duration
are necessary for the CSS animation
property to work as intended.
Note: for the shorthand to work properly, make sure you list the values in the same order as listed above.
Naming the animation
The CSS animation-name
property sets a particular animation to be applied to the chosen element:
.animated {
-webkit-animation-name: animation; /* Opera, Chrome, Safari */
animation-name: animation;
}
The syntax for this CSS animation property is simple:
animation-name: value;
The value can be either none
which represents no keyframes, or a custom name used to identify the animation. To create one, you can use letters (mind case-sensitivity), numbers, underscores and dashes.
Specifying the duration
To define the length of the animation cycle, use the CSS animation-duration
property:
.animated {
-webkit-animation-duration: 2s; /* Opera, Chrome, Safari */
animation-duration: 2s;
}
The syntax for CSS animation-duration
is simple – you just need to define the preferred duration in seconds:
animation-duration: value;
Note: if you don't define the duration for the animation, it will not run at all, as the default value is 0s.
Manipulating the animation pace
The CSS animation-timing-function
defines the pace of your animation:
animation-timing-function: value;
To make the changes smoother, a speed curve is used. If you are familiar with computer graphics, you might already know what a Bezier Curve means. Basically, it is a curve model defined by four points. When writing the animation-timing-function
property, you can define custom values for your curve or choose predefined options:
Value | Description |
---|---|
cubic‑bezier(n,n,n,n) | Different speeds at four parts of the animation defined by custom values in the range from 0 to 1 |
ease | The default value. Increases towards the middle, then slows back down |
linear | Keeps a stable pace |
ease-out | Starts quickly and slows down |
ease-in | Starts slowly and speeds up |
ease-in-out | Transitions slowly, speeds up and slows down again |
Another way to define the pace is by using steps:
animation-timing-function: steps(x, term);
In this kind of syntax, x
represents the number of steps for the animation. Each of them will be shown for the same amount of time. The term
defines the way it behaves:
Term | Meaning |
---|---|
jump-start | The first jump happens at the very start of the animation |
start | The first jump happens at the very start of the animation |
jump-end | The last jump happens at the very end of the animation |
end | The last jump happens at the very end of the animation |
jump-both | Pauses are added at the very start and the very end of the animation |
jump-none | There are no jumps at the very start and the very end of the animation |
If you only need to define one step, you can use step-start
instead of steps(1, jump-start)
and step-end
for steps(1, jump-end)
:
.step-start {
animation-timing-function: step-start;
}
.step-end {
animation-timing-function: step-end;
}
Defining the CSS animation delay
The CSS animation-delay
property can be used to specify the delay before an animation starts:
.animated {
-webkit-animation-delay: 3s; /* Opera, Chrome, Safari */
animation-delay: 3s;
}
The syntax is very straightforward – you just need to define the CSS animation delay in seconds:
animation-delay: value;
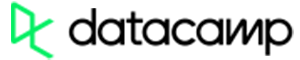
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
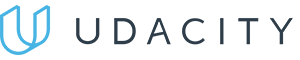
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
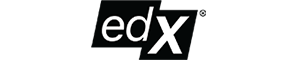
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Specifying the number of animation cycles
The CSS animation-iteration-count
property defines how many times an animation should normally play:
The syntax for this property is simple:
animation-iteration-count: value;
You can either define the number of times or use the keyword infinite
to make the animation run indefinitely. The default value for this property is 1
.
Modifying animation direction
To make a certain animation play in reverse or alternate cycles, use the CSS animation-direction
property:
div {
-webkit-animation-direction: alternate; /* Chrome, Safari, Opera */
animation-direction: alternate;
}
To use this property, you need to define one of the four available values:
animation-direction: value;
Value | Description |
---|---|
normal | Default. The animation runs normally |
reverse | The animation runs in reverse. Timing functions are reversed as well |
alternate | The animation runs normally, then keeps reversing the direction with each new cycle |
alternate-reverse | The animation runs in reverse, then keeps reversing the direction with each new cycle |
Note: the Safari browsers does not support reverse and alternate-reverse.
Styling an inactive animation
The CSS animation-fill-mode
property is used to set the style for an element while the animation is not active (finished or delayed):
.animated {
-webkit-animation-fill-mode: both; /* Opera, Chrome, Safari */
animation-fill-mode: forwards;
}
Typically, animations do not affect the element until they have finished playing. This CSS animation property can override such behavior.
The CSS animation-fill-mode
property accepts four values:
animation-fill-mode: value;
Value | Description |
---|---|
none | The default value. An animation has no styles applied when it's not running. |
forwards | The animation keeps the values of the last keyframe after it finishes running. |
backwards | The animation has the values of the first keyframe before it starts running. |
both | The animation will follow the rules applied by both forwards and backwards. |
Is the animation running?
The CSS animation-play-state
property defines whether the animation is running:
animation-play-state: value;
This CSS animation property accepts two values:
Value | Description |
---|---|
running | The animation is running |
paused | The animation is paused |
Tip: start learning animations with simple ones, such as CSS fade in and CSS fade out. It will also make creating sequences easier.
Browser support
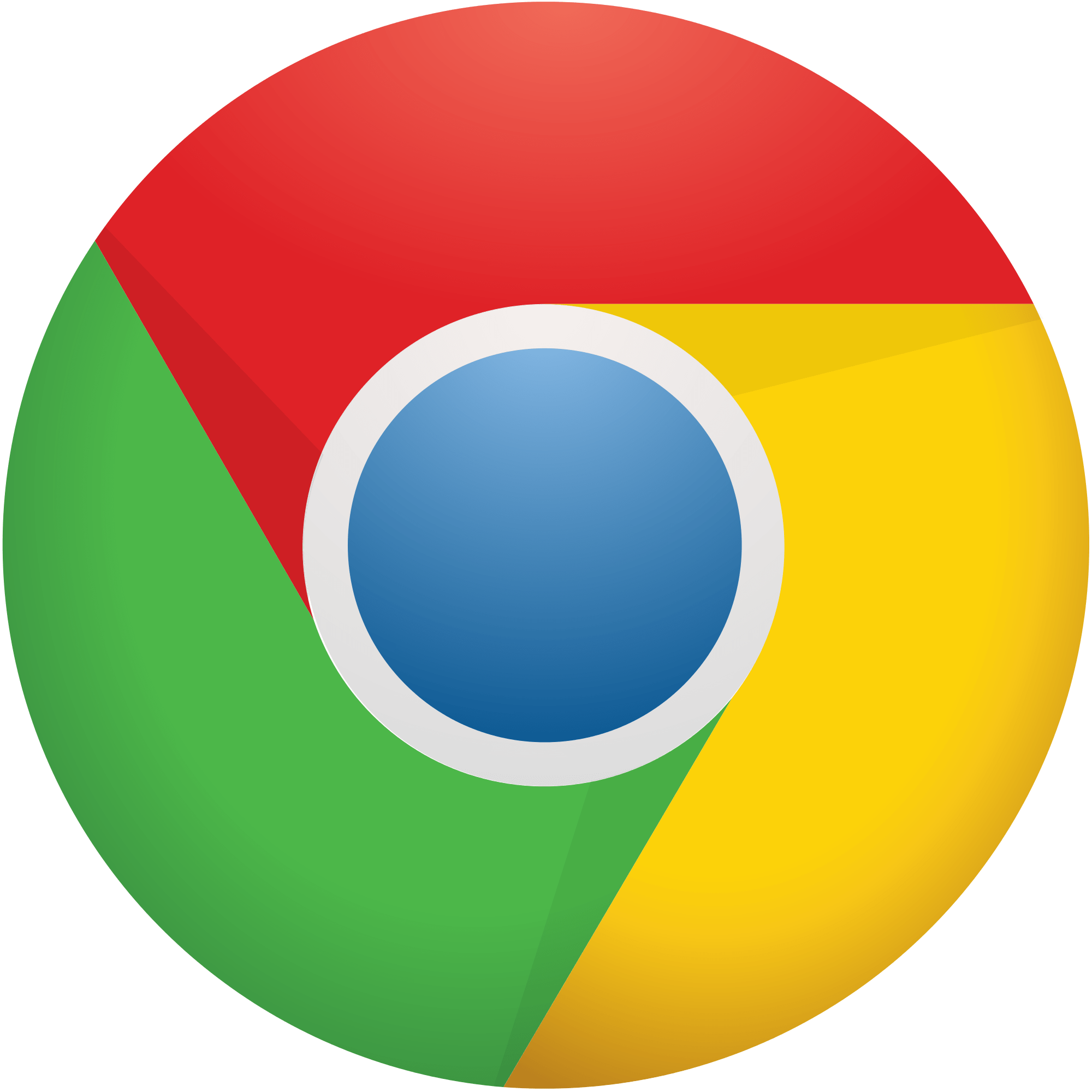
Chrome
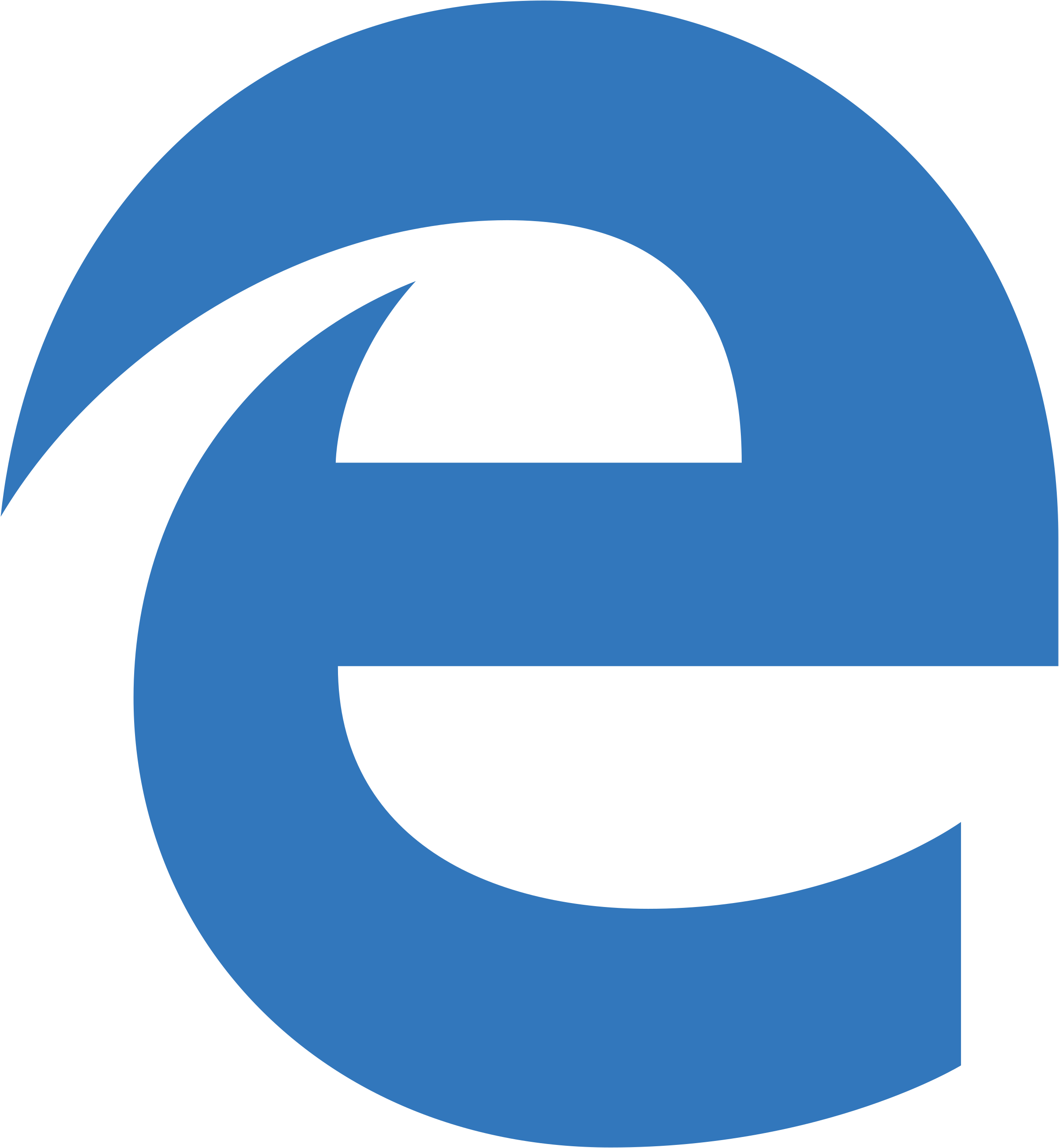
Edge
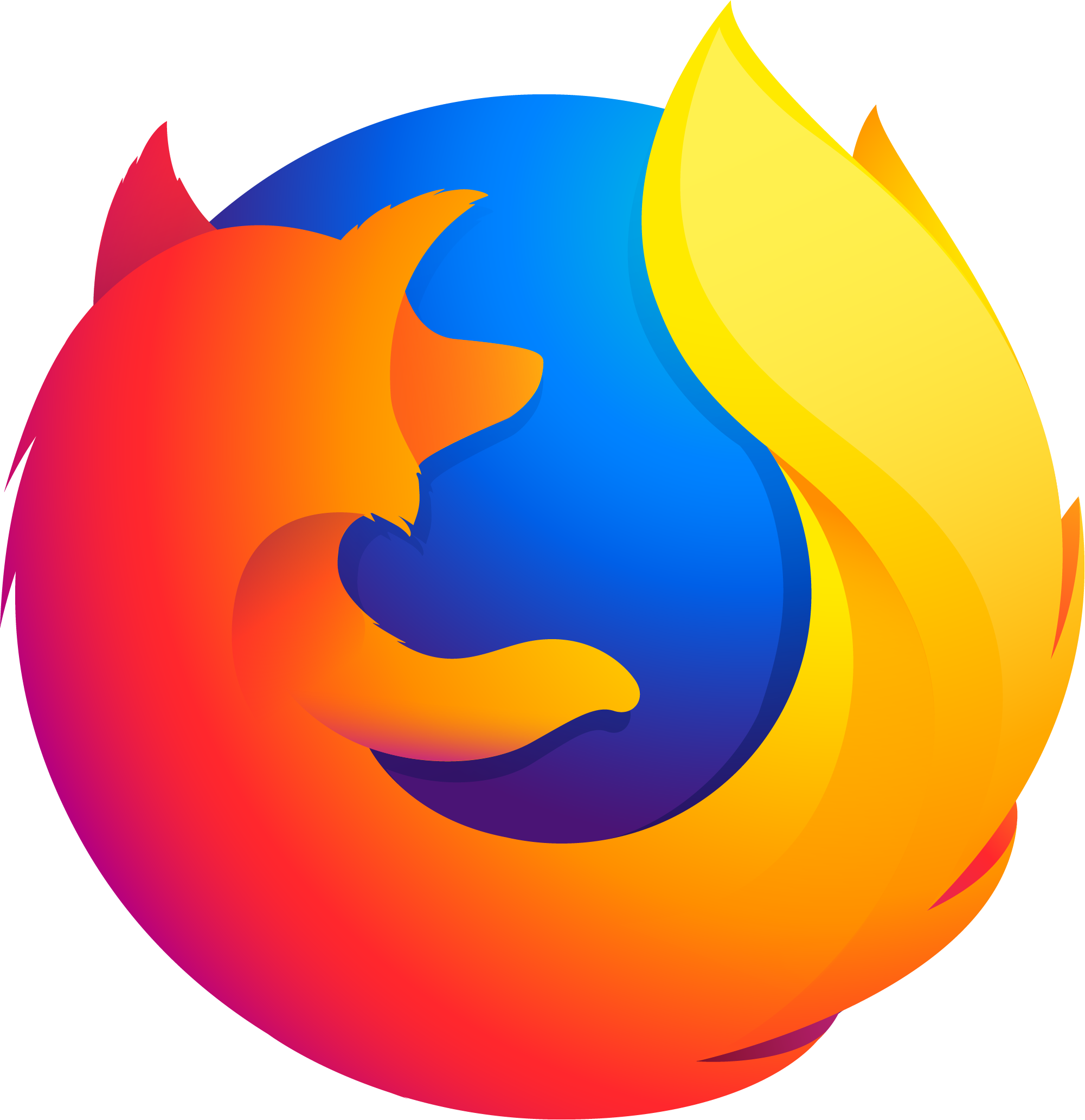
Firefox
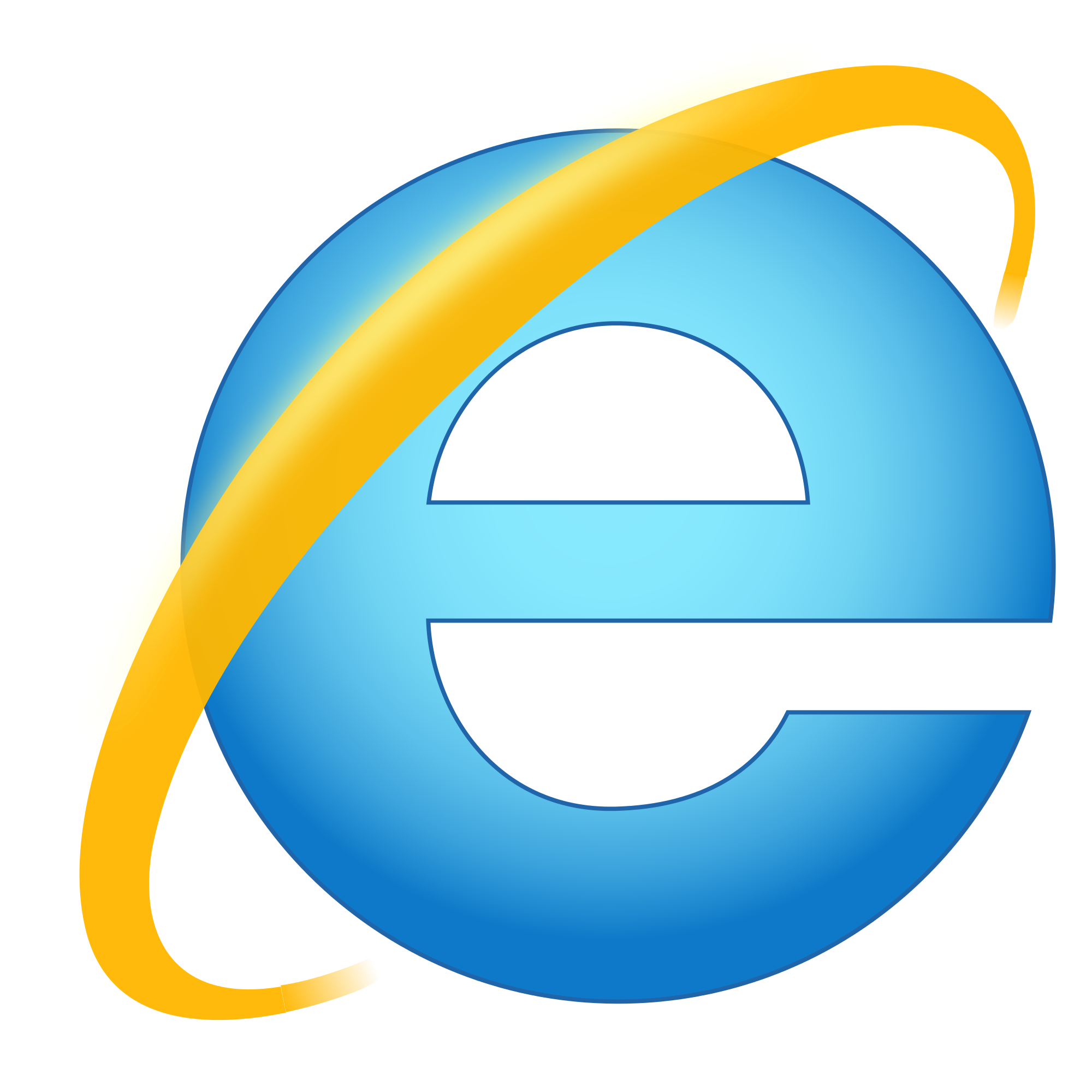
IE
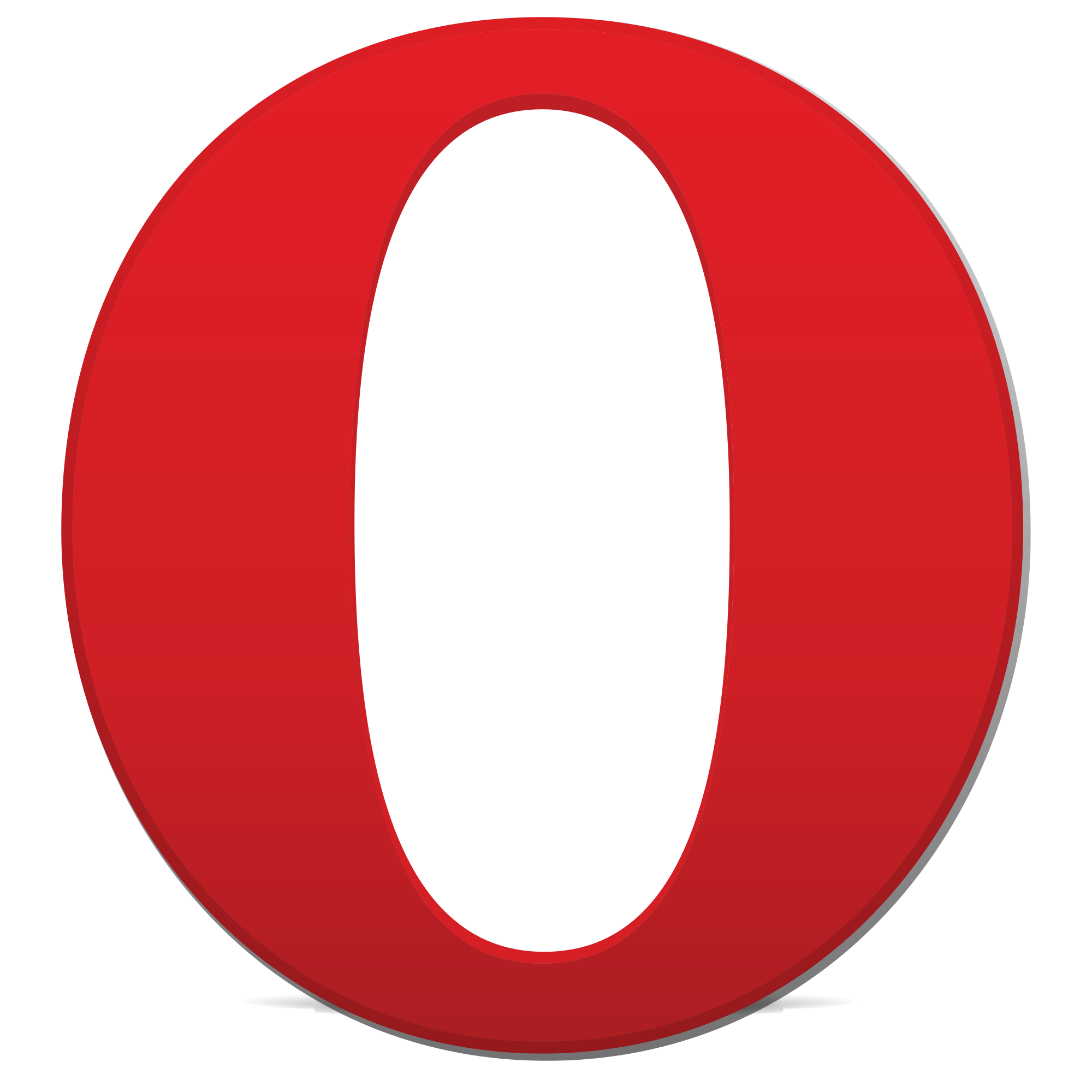
Opera
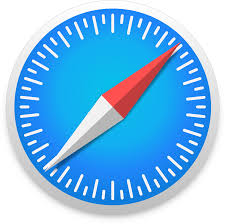
Safari
Mobile browser support
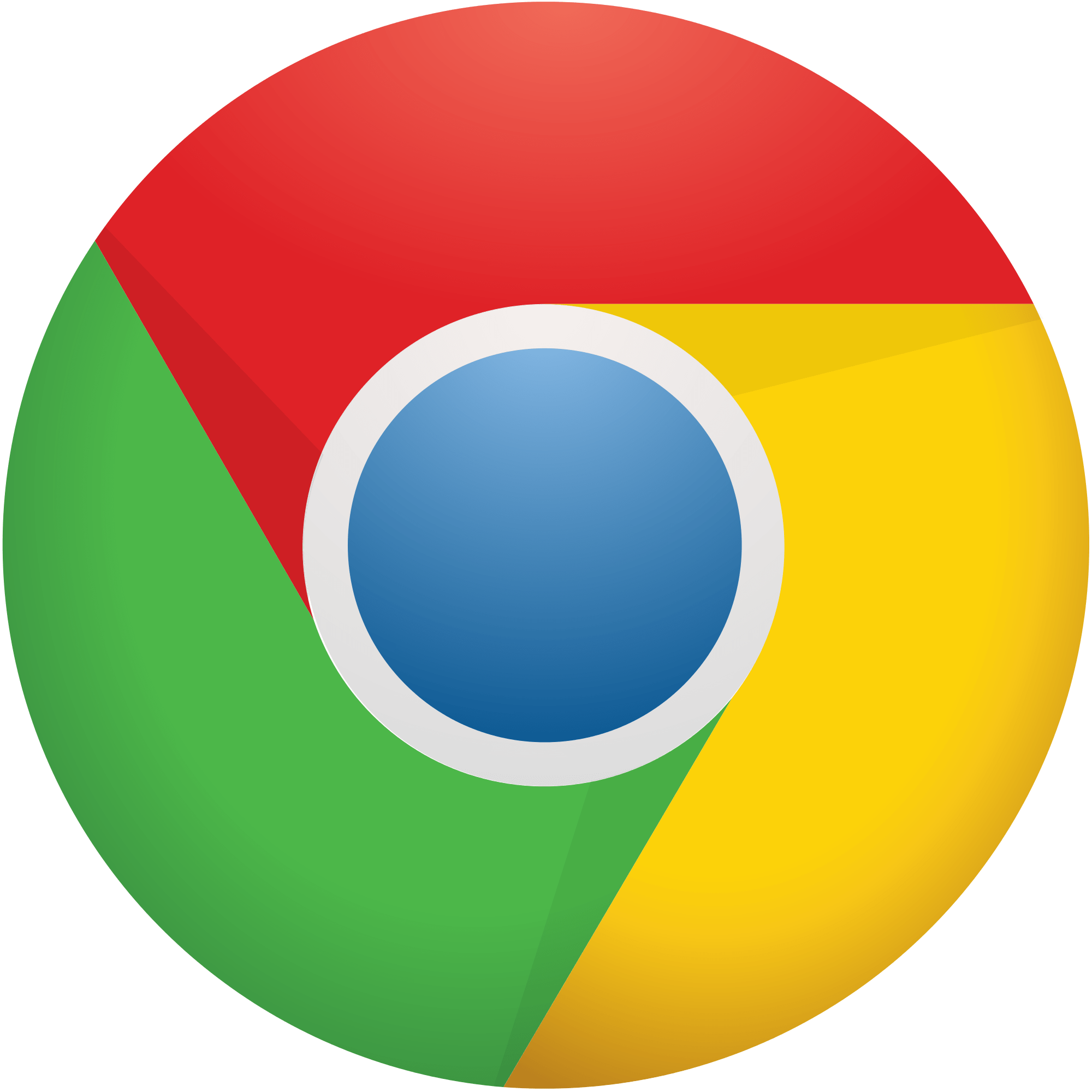
Chrome
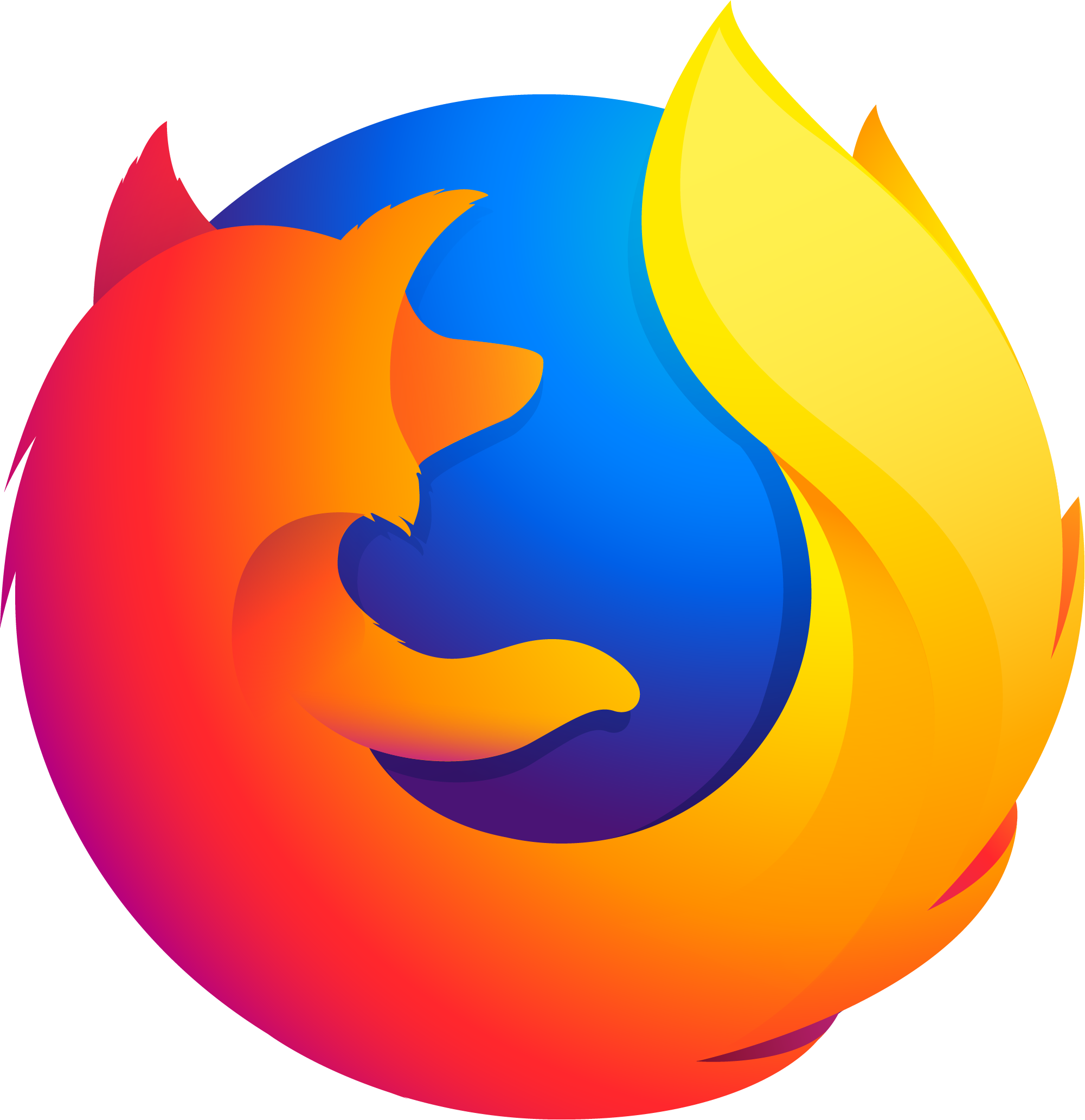
Firefox
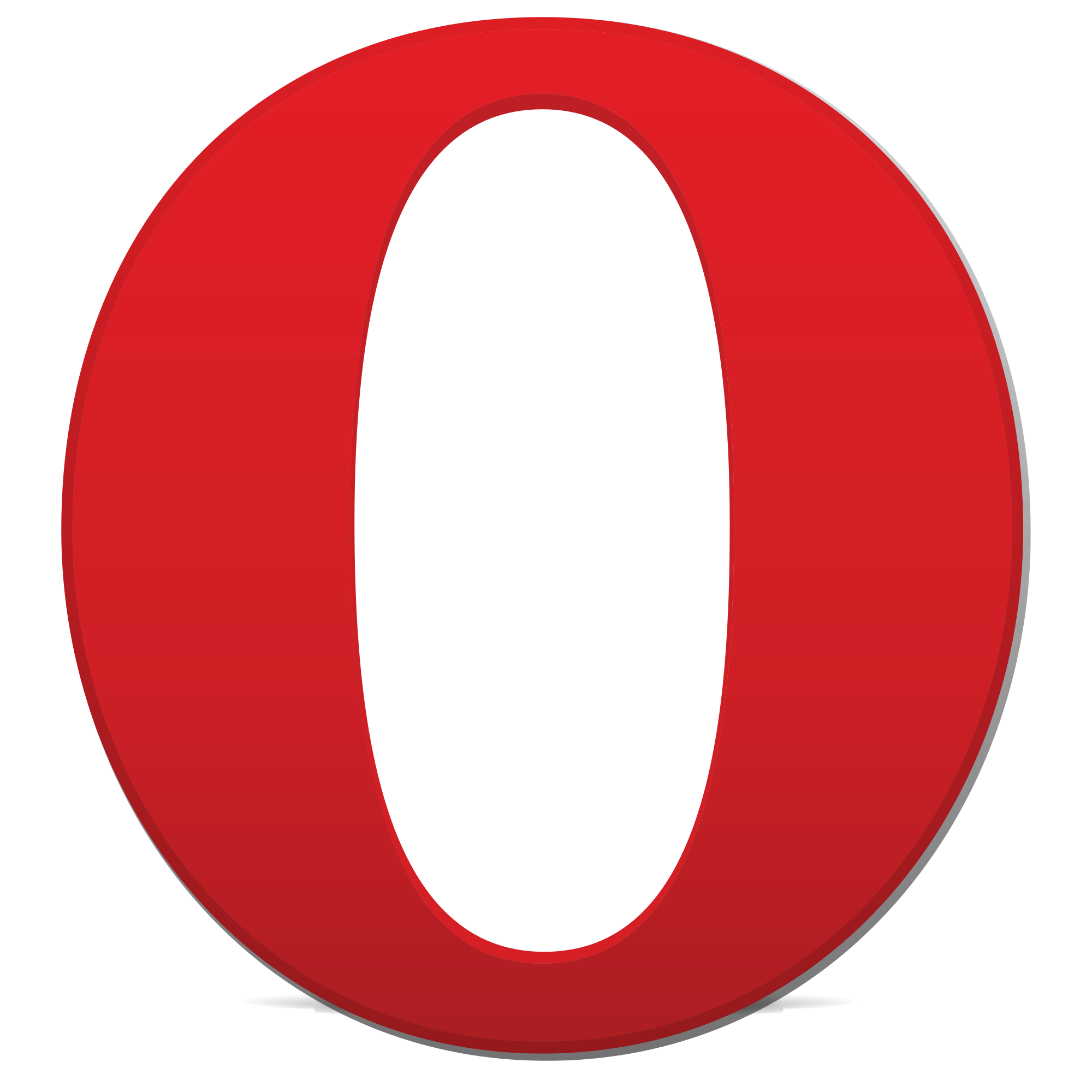
Opera
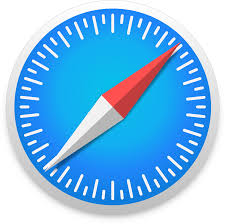