Tooltip text
Tooltip text
Tooltip text
Tooltip text
TL;DR – CSS tooltip refers to a popup message that appears after a mouse hover, or when elements get keyboard focus. It disappears on mouse out, or when the element loses keyboard focus.
Contents
Creating Tooltips With CSS
It is possible to create a pure CSS tooltip for an element. However, the first step is making an HTML tooltip by assigning a class to the element that will have the tooltip.
Then, you use the styling properties of CSS. The tooltip presented in the following example has the position, color, and display properties.
.tooltip { /* Container for our tooltip */
position: relative;
display: inline-block;
}
.tooltip .tooltiptext { /* This is for the tooltip text */
visibility: hidden;
width: 100px;
background-color: #8512d5;
color: white;
text-align: center;
padding: 10px;
border-radius: 10px; /* This defines tooltip text position */
position: absolute;
z-index: 1;
}
.tooltip:hover .tooltiptext { /* Makes tooltip text visible when text is hovered on */
visibility: visible;
}
The text of the tooltip is inside a <span> element with a tooltiptext
class.
Note: tooltips are for setting additional information about a topic when users hover the mouse pointer over styled elements.
Steps to Follow to Create a Pure CSS Tooltip
With HTML, we only add the elements with text. CSS sets the position, color and other properties for the tooltip.
- We set the
position
torelative
to the element with thetooltip
class. - We position the text inside the tooltip with
position: absolute
. - The element with the
tooltiptext
class has the text inside the tooltip. It is hidden until:hover
triggers. - CSS styles the HTML tooltip by indicating its width, and background-color.
- The text inside the tooltip is centered and has specific padding settings.
- The border-radius rounds the corners of the HTML tooltip.
Positioning
You can position a CSS tooltip on one of the four sides of an HTML element.
Right Tooltip
Tooltip text
The example below places the tooltip to the right side of the hoverable text by using the left
property with the position: relative
(to move it right).
Tip: we use top: -5px to move the CSS tooltip to the same height as the container.
By increasing the padding
, you might need to increase the value of the top
property to ensure it stays centered vertically.
Left Tooltip
Tooltip text
The creation of the HTML tooltip on the left side involves the use of right
with the position: relative
.
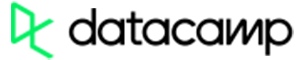
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
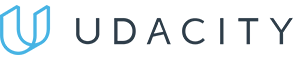
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
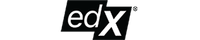
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Top Tooltip
Tooltip text
The following example adds the HTML tooltip at the top of the element:
.tooltip .tooltiptext {
width: 100px;
bottom: 100%;
left: 50%;
margin-left: -50px; /* This makes the margin half of the width to the center */
}
Note: we use the margin-left property with -60 to center the tooltip.
Tooltip text
Bottom Tooltip
Here is an example of a bottom CSS tooltip:
.tooltip .tooltiptext {
width: 100px;
top: 100%;
left: 50%;
margin-left: -50px; /* This makes the margin half of the width to the center */
}
Tip: do not forget to include the margin-left and set its value to -60 to center the tooltip.
Creating an Arrow
You can create arrows for your tooltips in CSS by combining the ::after
pseudo-element with an empty content
property. As a result, the tooltip resembles a speech bubble.
.tooltip .tooltiptext::after {
content: " ";
position: absolute;
top: 100%; /* This will position the arrow at the bottom of the tooltip */
left: 50%;
margin-left: -10px;
border-width: 10px;
border-style: solid;
border-color: black transparent transparent transparent; /* This will make the top border black*/
}
The example above follows these steps to create an arrow for a tooltip positioned on the bottom:
- There are no unique properties for creating arrows for tooltips. Therefore, we will use the border property.
- We need to position the arrow at the bottom by setting
top: 100%
. - The
left:50%
part of code centers the tooltip. - The
border-width
sets the size of the arrow. If you change the size, you also need to modify themargin-left
to keep the arrow centered. - The
border-color
sets the color of the arrow. You should only define the top border and leave other borders transparent. - You must set the
border-style
to define the way the border looks. The example uses thesolid
value.
The following example shows how you create an arrow for the tooltip positioned at the top:
.tooltip .tooltiptext::after {
content: " ";
position: absolute;
bottom: 100%; /* This will position the arrow at the top of the tooltip */
left: 50%;
margin-left: -10px;
border-width: 10px;
border-style: solid;
border-color: transparent transparent black transparent; /* This will make the top border black */
}
The below example demonstrates how you can add an arrow to the tooltip on the left side:
.tooltip .tooltiptext::after {
content: " ";
position: absolute;
top: 50%;
right: 100%; /* This will position the arrow on the left of the tooltip */
margin-top: -10px;
border-width: 10px;
border-style: solid;
border-color: transparent black transparent transparent; /* This will make the right border black*/
}
The below example shows how you can add an arrow to the tooltip on the right side:
.tooltip .tooltiptext::after {
content: " ";
position: absolute;
top: 50%;
left: 100%; /* This will position the arrow on the right of the tooltip */
margin-top: -10px;
border-width: 10px;
border-style: solid;
border-color: transparent transparent transparent black; /* This will make the left border black*/
}
Fade In Animation
Tooltip text
Without additional properties, a tooltip box appears suddenly. However, the transition and opacity styling properties make the tooltip fade in gradually.
In the example, we create a smooth fade-in effect:
.tooltip .tooltiptext {
opacity: 0; /* This will make the tooltip text invisible when it's not being hovered on */
transition: opacity 3s;
}
.tooltip:hover .tooltiptext {
opacity: 1;
} /* This will make it fade in when it's hovered on */
- We need to set
opacity: 0
for when the tooltip is hidden. - The
transition
property should indicate the duration of the animation. - We need to add
opacity: 1
to the tooltip for when:hover
triggers.
CSS Tooltip: Useful Tips
- One of the concerns of the use of tooltips is that users of mobile devices might be unable to use this feature.
- You can create tooltips by using the resources of Bootstrap.