TL;DR — The CSS transform property visually controls elements and lets you scale, rotate, skew, and translate elements.
Contents
Using the transform property
By using CSS transform
property, you can make elements change their appearance. It works with most of the HTML elements, except elements that are not controlled by the box model. Additionally, transform
cannot work with non-replaced inline, table-column-group, and table-column boxes.
The transform
property accepts only two values: one of the specific transform functions or none
(indicates that transform does not apply).
Transform: translate
The CSS transform: translate()
property is for moving elements to the left or right side, or up and down. It accepts two values:
- One value means that element will be moved up and down or side-to-side. Remember that negative values move elements to the left, positive ones to the right.
- The second value pushes the element down. Negative values move elements up, while positive ones move them down.
The following example moves an HTML element to the right and down with the two values:
div {
width: 80px;
height: 80px;
background-color: green;
}
.example1 {
background-color: purple;
color: white;
border-radius: 5px;
transform: translate(20px, 50px);
}
It is also possible to move elements along the horizontal or vertical axis. The translateY
moves elements vertically, while translateY
pushes them horizontally.
div {
width: 80px;
height: 80px;
background-color: green;
}
.example1 {
transform: translateY(130px);
background-color: purple;
}
You also can move elements in the 3D space by using CSS transform: translate3d
or the translateZ
. These functions create an effect that elements move closer or further away from the user.
div {
width: 100px;
height: 100px;
background-color: green;
}
.example1 {
transform: perspective(500px) translate3d(50px, 10px, 3px);
background-color: pink;
}
Transform: scale
The CSS transform: scale()
property is for scaling elements.
If one value is specified, the element scales the specified number of times its original size. When you set two values, the element stretches horizontally according to the first value, and vertically by the second one.
The following example resizes an element six times its normal size:
.example1 {
background-color: purple;
height: 100px;
width: 100px;
transform: scale(6);
}
Note: it is also possible to use scale without the transform property. However, this is experimental and does not have the best browser support.
Similarly to translate
, the CSS transform: scale
is a shorthand for scaleX
(for resizing horizontally) and scaleY
(for resizing vertically).
The following example uses the scaleY
to resize an element:
.example1 {
background-color: purple;
height: 100px;
width: 100px;
transform: scaleY(3);
}
You can also use CSS transform: scale3d
or the scaleZ
to resize an element in 3D space.
Transform: rotate
The CSS transform: rotate()
is for making elements move around a fixed point. By default, it rotates around the center of the element.
The following example creates an animation of rotation:
div {
display: inline-block;
background-color: purple;
height: 200px;
width: 150px;
padding: 1px;
margin: 5px;
border-radius: 100%;
animation: roll 5s infinite;
transform: rotate(30deg);
}
@keyframes roll {
0% {
transform: rotate(0);
}
100% {
transform: rotate(360deg);
}
}
rotateX()
is for making elements rotate around the horizontal axis. The function does not deform the element. It works the same as rotate3d(1,0,0,a)
.
The rotateX()
works with angle
values. If they are positive, the element moves clockwise. Negative values make the element move in the opposite direction.
div {
width: 100px;
height: 100px;
background-color: green;
}
.example1 {
transform: rotateX(45deg);
background-color: purple;
}
rotateY()
is for rotating an element around its vertical axis. It works the same as rotateX
by accepting angle
values and moving clockwise if the values are positive.
div {
width: 80px;
height: 80px;
background-color: green;
}
.example1 {
transform: rotateX(45deg);
background-color: purple;
}
The CSS transform: rotateZ()
is for rotating an element around the Z-axis (3D space).
Note: the Z-axis represents the depth and is perpendicular to both X an Y-axis.
The element is rotated at the angle you specify in the brackets.
div {
-webkit-transform: rotateZ(135deg); /* Safari */
transform: rotateZ(135deg);
}
Transform: skew
The CSS transform: skewX
property tilts elements horizontally. The skewY
tilts elements vertically.
Note: a shorthand for these two functions does not exist. You have to use both if you need to skew an element both vertically and horizontally.
The following example uses CSS transform: skewX
and skewY
functions to show their difference:
div {
width: 80px;
height: 80px;
background-color: skyblue;
margin-right: 15px;
margin-left: 15px;
}
.example1 {
transform: skewX(10deg);
background-color: pink;
}
.example2 {
transform: skewY(10deg);
background-color:green;
}
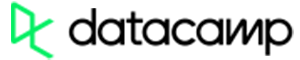
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
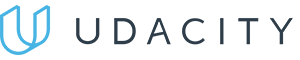
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
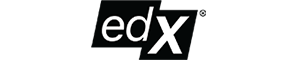
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
transform-origin
The CSS transform-origin
property indicates the point from which the element should be transformed. For instance, the default origin of all transform functions is the center.
CSS transform-origin
works with two keywords indicating the direction (for instance, left and right). It also uses length values or a combination of keywords and length. Three values indicate that element will have CSS 3d transform effect.
The following code example changes the default origin and starts the transformation from a different point:
div {
display: inline-block;
background-color: purple;
height: 200px;
width: 150px;
padding: 1px;
margin: 5px;
border-radius: 100%;
animation: roll 5s infinite;
transform: rotate(30deg);
transform-origin: 50px 50px;
}
transform-style
The CSS transform-style
property indicates whether the children of elements should be in the 3D space or flattened. In other words, you specify whether the CSS 3d transform effect passes on to children or whether they appear as regular HTML elements.
The following code example shows how the preserve-3d
value makes children remain in the 3D space, while flat
value makes them look like normal elements:
div {
display: inline-block;
background-color: purple;
height: 200px;
width: 150px;
padding: 1px;
margin: 5px;
border-radius: 100%;
animation: roll 5s infinite;
transform: rotate(30deg);
transform-origin: 50px 50px;
transform-style: preserve-3d;
}
transform-box
The CSS transform-box
indicates the layout box that will relate to the transform
and transform-origin
properties. It can have the following values:
view-box
makes the closest SVG viewport become the reference box.fill-box
makes the object bounding box become the reference box.border-box
makes the border box become the reference box.
Warning: this is an experimental property, meaning that it does not have the best browser support.
backface-visibility
The backface-visibility
is related to the CSS 3D transform effect. It indicates whether the back face of an element should be visible
or hidden
. However, this is an experimental property, meaning that some browsers have difficulty presenting it correctly.
This code example shows the syntax of backface-visibility
:
.example1 {
-webkit-transform: rotateY(180deg);
-moz-transform: rotateY(180deg);
-ms-transform: rotateY(180deg);
-webkit-backface-visibility: visible;
-moz-backface-visibility: visible;
-ms-backface-visibility: visible;
}
.example2 {
-webkit-backface-visibility: hidden;
-moz-backface-visibility: hidden;
-ms-backface-visibility: hidden;
}
Note: the backface-visibility should always be written as
-webkit-backface-visibility
.
perspective
The CSS perspective
property sets an element 3D space by indicating the distance between the Z plane and users. Smaller values create a more noticeable effect since you get closer from the Z plane. Go with bigger values to generate a more gentle effect.
When you use the perspective
together with CSS transform
, the property sets the 3D space, while the perspective
property alone makes the parent share the 3D space with its children.
The following example shows the perspective
property in action:
.parent.perspective {
perspective: 70em;
}
.parent.perspective .child {
transform: rotateX(70deg);
background: rebeccapurple;
}
perspective-origin
The CSS perspective-origin
is for setting the position at which the user sees. The property has no effect if you do not set perspective
as well. Additionally, it needs to be assigned to the parent element.
The following code example shows how perspective-origin
works:
.example1 { perspective-origin: 15% 65%; }
.example2 { perspective-origin: 15px 35px; }
.example3 { perspective-origin: left bottom; }