TL;DR â The JavaScript download method allows you to declare a unique function for downloading files without contacting the server and lets you hide the file path from users.
Contents
What are automatic downloads with JavaScript?
Automatic file download with JavaScript is a method that allows you to retrieve a file directly from the URL by declaring a JavaScript function.
It is done without sending an action request to a server. You can use this method on browsers that support HTML5.
Note: automatic downloads allow you to build a secure download link that prevents users from seeing the file path.
You can also encrypt the download link by setting a password and expiry date on it.
In the past, popular browsers have made automatic downloads difficult to execute due to safety concerns. With the HTML5 and JavaScript download method, this is no longer an issue.
Making JavaScript download files without the server
Here is the syntax for downloading a file directly from browsers:
function download(filename, text) {
var element = document.createElement('a');
element.setAttribute('href', 'data:text/plain;charset=utf-8,' + encodeURIComponent(text));
element.setAttribute('download', filename);
element.style.display = 'none';
document.body.appendChild(element);
element.click();
document.body.removeChild(element);
}
// Start file download.
document.getElementById("dwn-btn").addEventListener("click", function(){
// Start the download of yournewfile.txt file with the content from the text area
var text = document.getElementById("text-val").value;
var filename = "yournewfile.txt";
download(filename, text);
}, false);
This way of making JavaScript download files allows users to retrieve the content provided in the text area. The file reaches the computer device as a simple .txt, opening in the standard text editor.
The declared function sets a download attribute where you get to name the file. The encodeURIComponent() function then encodes the content. The click() method prompts the download process to start as you click the download button.
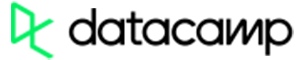
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
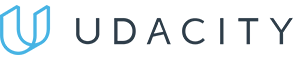
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
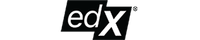
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Using FileSaver.js to download files on the client-side
There is another option for downloading files without contacting the server. This time, we are using a JavaScript library called FileSaver.js. The example below presents the syntax to implement the saveAs()
FileSaver interface:
function download() {
var save = document.getElementById("example").value;
var blob = new Blob([save], {
type: "text/plain;charset=utf-8"
});
saveAs(blob, "yournewfile.txt");
}
Note: this option allows you to implement the
saveAs()
FileSaver interface in browsers that donât support it. It sets the file to a Blob value.
FileSaver.js is a solid solution for downloading files on the client-side instead of involving the server-side. For instance, it is useful for preventing sensitive information from being sent to external servers.
What is a Blob?
Blob, which stands for Binary Large Object, represents data that doesnât support JavaScript-native format. It includes but is not limited to multimedia objects, programs, and code snippets.
Remember: as Blob has size limitations based on the supported browser, FileSaver.js is only suitable for small or medium sized files (500-800 MiB max).
See the table below to check the compatibility of FileSaver.js in different browsers:
Browser | Builds as | Filenames | Max Blob Size | Dependencies |
---|---|---|---|---|
Firefox 20+ | Blob | Yes | 800 MiB | None |
Firefox < 20 | data: URI | No | n/a | Blob.js |
Chrome | Blob | Yes | 500 MiB | None |
Chrome for Android | Blob | Yes | 500 MiB | None |
Edge | Blob | Yes | Unknown | None |
IE 10 | Blob | Yes | 600 MiB | None |
Opera 15+ | Blob | Yes | 500 MiB | None |
Opera < 15+ | data: URI | No | n/a | Blob.js |
Safari 6.1+ | Blob | No | Unknown | None |
Safari < 6 | data: URI | No | n/a | Blob.js |
The following code snippet checks whether a browser supports Blob objects:
try {
var isFileSaverSupported = !!new Blob;
} catch (e) {}
JavaScript download: useful tips
- In some cases, instead of downloading blobs, Safari 6.1+ users will open them. Then, they would have to press a combination of ? + S on their keyboard to save the opened file.
- As FileSaver.js can only support small to medium-sized files, you can use StreamSaver.js for large files.