In this chapter, we will be covering methods used to add jQuery content. After you grasp their key differences, learning will be a breeze. You will learn how to make jQuery add child to a parent element and perform other similar additions.
Contents
jQuery Add Element: Main Tips
- jQuery has useful methods and selectors which allow you to manipulate DOM more conveniently than by simply using JavaScript.
- Using jQuery methods, you can add elements and content both inside and outside HTML tags of the elements.
- To make jQuery add child to an element, use
.prepend()
for the first child and.append()
for the last.
Available Methods
There are four methods that are used to add jQuery content:
.append()
- insert content at the end of selected element (inside its HTML tags).prepend()
- insert content at the start of selected element (inside its HTML tags).after()
- insert content after the selected element (outside its HTML tags).before()
- insert content before the selected element (outside its HTML tags)
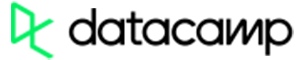
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
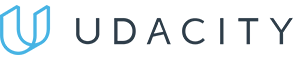
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
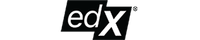
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
.append() or .prepend()
The .append()
and .prepend()
methods both follow this simple syntax:
$(selector).append | prepend(htmlContent, [htmlContent])
htmlContent
can be a string, but it can also be a variable, and you can add as many of them as needed (separated by commas). This allows several elements to be added using a single .append()
or .prepend()
statement:
$("#btn1").click(() => {
$("p").prepend("<strong>Added to the front</strong>. ");
});
$("#btn2").click(() => {
$("ol").prepend("<li>Added to the top</li>");
});
$("#btn3").click(() => {
$("p").append(" <strong>Added to the back</strong>.");
});
$("#btn4").click(() => {
$("ol").append("<li>Added to the back</li>");
});
.after() and .before()
The .after()
and .before()
methods place new content outside the tags of the selected element. Both of them follow syntax similar to the append and prepend statements:
$(selector).after | before(htmlContent, [htmlContent])
These methods can be used to add multiple elements as well. However, unlike in the previous example, if you were to select the <body> element, the newly added elements wouldn't appear anywhere since they would end up outside of the body of the web page:
$("#btn1").click(() => {
$("img").before("<strong>This text is on the left side</strong>");
});
$("#btn2").click(() => {
$("img").after("<strong>This text is on the right side</strong>");
});
Note: If you need to make jQuery create elements to use first, don't forget to start with the document ready event.
jQuery Add Element: Summary
- Among other ways to conveniently modify and understand DOM information, jQuery offers a handy functionality that lets you add content to HTML elements.
- By applying
.prepend()
or.append()
, you can make jQuery add child to an HTML DOM element. - You can use these methods to add jQuery, JavaScript, or HTML made elements both inside and outside of their tags.