Contents
jQuery mouseenter: Main Tips
- The jQuery
.mouseenter()
adds an event handler, running a function when the mouseenter event occurs, or triggers the event handler. - The
mouseenter
event happens once a mouse pointer enters elements. - Unlike an otherwise similar .mouseover() method,
.mouseenter()
doesn't react to event bubbling.
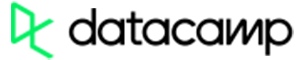
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
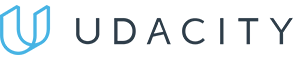
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
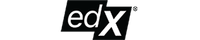
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Usage of Syntax of .mouseenter()
The .mouseenter()
method in jQuery attaches an event handler, invoking a function when the mouseenter
event occurs. The function can also trigger the handler for said event.
In the example below, a paragraph of text changes background color to red when a jQuery mouse enter event is detected:
$("div").mouseenter(() => {
$("div").css("background-color", "red");
});
Follow this syntax to trigger the mouseenter
event handler:
$("selector").mouseenter();
Attach the event handler by using this syntax:
$("selector").mouseenter(function);
Event Bubbling with .mouseenter()
In the example below, you can see the difference between jQuery .mouseenter()
and .mouseover() methods:
var i = 0;
$( "div.overout" )
.mouseover(function() {
$( "p:first", this ).text( "mouse over" );
$( "p:last", this ).text( ++i );
})
.mouseout(function() {
$( "p:first", this ).text( "mouse out" );
});
var n = 0;
$( "div.enterleave" )
.mouseenter(function() {
$( "p:first", this ).text( "mouse enter" );
$( "p:last", this ).text( ++n );
})
.mouseleave(function() {
$( "p:first", this ).text( "mouse leave" );
});
As you can see, unlike .mouseover()
, .mouseenter()
does not support event bubbling. This means the event only triggers its handler when the cursor enters the element it is applied to, not caring about its parents or children.