Contents
SQL IN Operator Introduced
In SQL, IN
is a logical operator that allows you to filter a set of values against a specific condition. It is usually used with the WHERE clause.
In the parentheses, you can define the exact values you need to be matched by the data entries:
SELECT column_demo(z)
FROM table_demo
WHERE column_demo IN (value1, value2, ...);
You can also use the SELECT statement to create a subquery that returns a list of values in a specified column:
SELECT column_demo(z)
FROM table_demo
WHERE column_demo IN (SELECT STATEMENT);
Demo Databases
In this tutorial, we will be using two demo tables for our data – Developers and Customers.
Developers
ID | Name | City | Country |
---|---|---|---|
1 | Tom Kurkutis | New York | USA |
2 | Ana Fernandez | London | UK |
3 | Antonio Indigo | Paris | France |
4 | Aarav Kaelin | Delhi | India |
5 | Andrew Tumota | Miami | USA |
6 | Basma Zlata | Miami | USA |
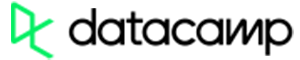
- Easy to use with a learn-by-doing approach
- Offers quality content
- Gamified in-browser coding experience
- The price matches the quality
- Suitable for learners ranging from beginner to advanced
- Free certificates of completion
- Focused on data science skills
- Flexible learning timetable
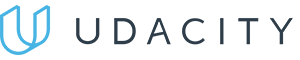
- Simplistic design (no unnecessary information)
- High-quality courses (even the free ones)
- Variety of features
- Nanodegree programs
- Suitable for enterprises
- Paid Certificates of completion
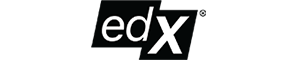
- A wide range of learning programs
- University-level courses
- Easy to navigate
- Verified certificates
- Free learning track available
- University-level courses
- Suitable for enterprises
- Verified certificates of completion
Customers
id | name | contact | address | city | postal_code | country |
---|---|---|---|---|---|---|
1 | Ben Choplinks | Ben Choplink | Obeesre Str. 51 | Rome | 11207 | Italy |
2 | Donald Rich | Donald Richario | Avda. de la Confgfstitución 4122 | Tallin | 17021 | Estonia |
3 | Lilly Smilkins | Lilly Smilkin | Matadsderos 2312 | Eguero | 14023 | Mexico |
4 | Brandinina | Tom Hitchins | 110 Hanegover Sq. | London | WB2 2DP | UK |
5 | Carizmos | Christiano Kerrys | Berguvsesvägen 9 | Lulea | S-968 43 | Sweden |
Examples of Using SQL IN()
The example below picks all the developers from USA, France and India:
SELECT * FROM Developers
WHERE Country IN ('USA', 'France', 'India');
For an opposite result, you can also use the NOT IN
operator in SQL. The code in this next example picks out only the developers who are not from those countries:
SELECT * FROM Developers
WHERE Country NOT IN ('USA', 'France', 'India');
Working with IN() in Multiple Tables
In the last example, we will try something a bit more complicated. The code you can see below uses both of our demo tables to pick all the developers whose countries match those of the customers:
SELECT * FROM Developers
WHERE Country IN (SELECT Country FROM Customers);
Instead of specifying the values for SQL IN()
, we include SELECT Country FROM Customers
within the parentheses. This means the SELECT statement will take the values from the Country
column in the Customers
table.